Most of us have uninstalled programs from our PCs. How have you done it? Can you recollect?
We’ve done it mostly using the Control Panel or in some cases, if dealing with more complex suites, their own uninstall process.
These options are convenient to remove a single program, they’re not scalable. Imagine for a moment you’re an IT admin and want to remove programs from multiple computers. Logging into each device and uninstalling a program isn’t the most productive option.
Thankfully, you can use PowerShell scripts to uninstall software. The advantage is you can send any number of networked PCs an uninstall command. This way, you can uninstall a program from thousands of PCs with just one script.
Sounds simple, right? Let’s jump in and see how to uninstall software using PowerShell.
What is Microsoft PowerShell?
Microsoft PowerShell is a task automation and configuration manager consisting of a scripting language and a command-line prompt. It comes with many built-in commands called cmdlets that perform a specific function or task. You can combine these cmdlets into a custom script to perform the desired task – automating complex or time-consuming tasks.
2 Ways to Uninstall Software Using PowerShell
You can uninstall software using PowerShell in 2 ways, the Uninstall() method and the Uninstall-Package command. Out of the two, the Uninstall() method is the most popular and the easiest option to remove well-known programs from a device.
The second option is the Uninstall-Package, it’s a good choice for hidden programs and ones PowerShell doesn’t identify.
Let’s start with the first option.
1. Uninstall Method
In this step-by-step guide, you’ll learn the commands to uninstall a software from a single computer. You can always use a For-each loop to extend it to a collection or array of PCs to iterate through every computer in the array and perform the same action.
Step 1: Get the List of Installed Applications
As a first step, get the list of applications installed on a computer. The PowerShell code for that is:
Get-WmiObject -Class Win32_Product | Select-Object -Property Name
Now, you may wonder what’s the need to get the list of installed applications? After all, you only need to remove one application from the computer. The catch is you must know the application’s exact name as PowerShell reads and displays it. For example, you may use the words “Microsoft Outlook” in your code to uninstall Outlook from a computer. What if it’s called “Microsoft Outlook 2019”? This mismatch will either throw an error message or the command will simply not execute. Avoid any confusion and see how PowerShell reads a software’s name and then, use this name exactly in your code to uninstall.
Here is an example, the image below shows how Get-WmiObject displays the installed programs’ list.
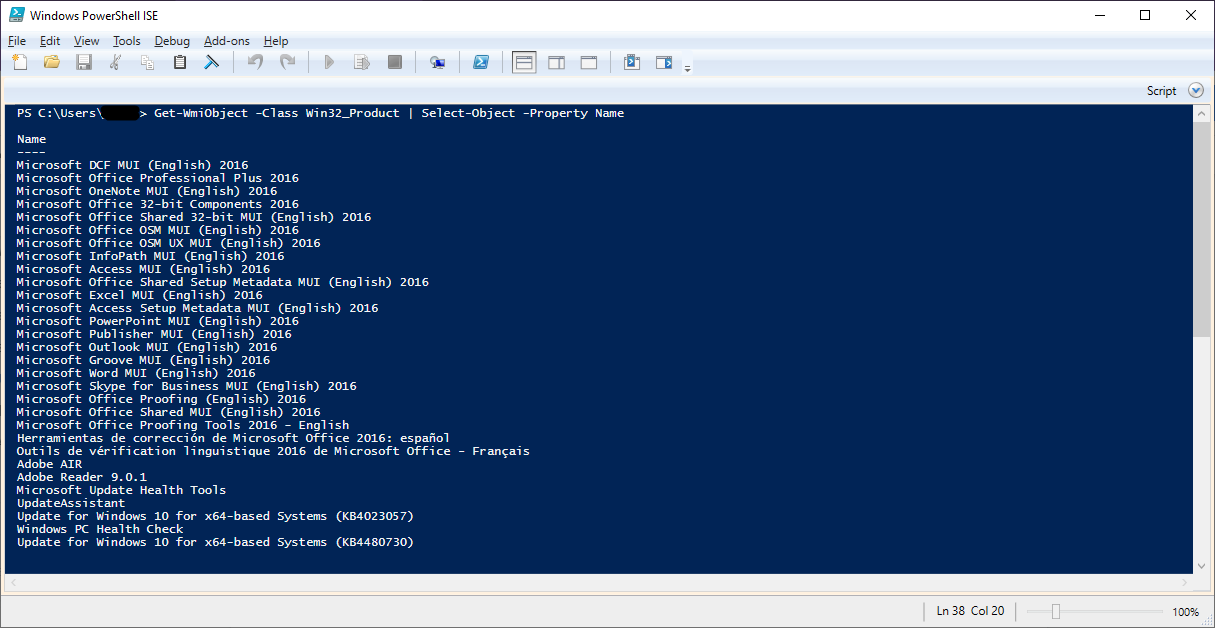
Step 2: Narrow the List
The programs’ list above can look overwhelming, even on devices with just a few installed programs. You can narrow it down using filters and regular expressions if you have an idea of your program’s name. In the above example, you can ask PowerShell to narrow the list to programs that contain the word “Outlook” as this is a unique word. If you use “Microsoft”, you can end up with a bigger list! In general, make sure you pick the expressions and words that have the best chance to help you find the program you want.
$MyProgram = Get-WmiObject -Class Win32_Product | Where-Object{$_.Name -eq “Outlook”}
This will narrow down the list to all the Microsoft Outlook versions installed in your system. From this list, pick the version you want to uninstall. If you have only one version installed, then nothing to pick, and MyProgram stores the value in the variable.
Step 3: Use the Uninstall Method
PowerShell comes with a built-in method called Uninstall(). Simply call this method on your program to uninstall it. In our above example, it’ll be
$MyProgram.uninstall()
This command will uninstall your program. You can also replace the variable $MyProgram with the actual program name.
This process is the easiest way to uninstall a program using PowerShell. That said, some hidden programs may exist and they won’t get listed with the Get-WmiObject command.
Open your Control Panel and see programs’ list on it to test this. Compare it with the list PowerShell displays and you’ll notice some aren’t included.
2. Use Uninstall-Package
Use this option if PowerShell doesn’t list your program. The code for this PowerShell command is,
Get-Package -Provider Programs -IncludeWindowsInstaller -Name “BackZilla”
To get PowerShell to display all the programs on the Control Panel, use an asterisk in place of the Name parameter. Note that if you’ve installed multiple versions, this command uninstalls only the latest version.
In the above example, it will be:
Uninstall-Package -Name BackupZilla 3.0
You can also choose to uninstall a specific version or pipe the output of Get-Package to Uninstall-Package. Here’s an example.
Get-Package -Name BackupZilla -RequiredVersion 2.0 | Uninstall-Package
Thus, this is how you can uninstall any program from a device, even if they’re hidden!
Comparison of Both Uninstall Methods
So, which of two should you choose? If Get-WmiObject displays a program you have, the Uninstall method is the easier choice. That said, if you have to create a custom script to remove programs across multiple computers, go for the Uninstall-Package.
Final Words
PowerShell scripts are convenient to remove a program across multiple devices. You can automate this process and save time and effort with just a few lines of code. You can uninstall software using PowerShell in 2 ways – the Get-WmiObject and Uninstall-Package. Out of the two, the Uninstall-package has more options and comes with wider access to normal and hidden programs while the Get-WmiObject command can only use the associated WMI classes.
Which of the two is better? It depends on the software you want to uninstall. If PowerShell’s Get-WmiObject recognizes it, go with this option as it’s easier. Otherwise, use Uninstall-Package.
Ref: https://techgenix.com/how-to-uninstall-software-using-powershell/